تولید خودکار برچسب برای تصاویر و توضیح محتوای آنها با استفاده از GPT-4-Vision #
این Notebook توضیح میدهد که چگونه میتوان از GPT-4-Vision برای برچسب زدن و توضیح تصاویر بهره برد. ما میتوانیم از تواناییهای GPT-4V استفاده کنیم تا تصاویر ورودی را همراه با اطلاعات تکمیلی در مورد آنها پردازش کند و برچسبها یا توضیحات مربوط به را خروجی دهد. سپس میتوان توضیحات تصویر را با استفاده از یک مدل زبانی (در این نوتبوک، ما از GPT-4-turbo استفاده خواهیم کرد) برای تولید توضیحات بیشتر اصلاح کرد.
تولید محتوای متنی از تصاویر میتواند در موارد متنوعی استفاده شود، به خصوص برای جستجوی میان تصاویر. در این Notebook جستجوی میان تصاویر را با استفاده از کلمات کلیدی تولید شده و توضیحات محصول - هم از ورودی متن و هم از ورودی تصویر - نشان خواهیم داد. به عنوان مثال، ما از مجموعهای از تصاویر مبلمان استفاده خواهیم کرد، آنها را با کلمات کلیدی مربوطه برچسب میزنیم و توضیحات کوتاه و توصیفی تولید میکنیم.
برای اجرای کدهای زیر ابتدا باید یک کلید API را از طریق پنل کاربری گیلاس تولید کنید. برای این کار ابتدا یک حساب کاربری جدید بسازید یا اگر صاحب حساب کاربری هستید وارد پنل کاربری خود شوید. سپس، به صفحه کلید API بروید و با کلیک روی دکمه “ساخت کلید API” یک کلید جدید برای دسترسی به Gilas API بسازید.
Setup
1from IPython.display import Image, display
2import pandas as pd
3from sklearn.metrics.pairwise import cosine_similarity
4import numpy as np
5from openai import OpenAI
6
7# Initializing OpenAI client
8client = OpenAI(
9 api_key=os.environ.get(("GILAS_API_KEY", "<کلید API خود را اینجا بسازید https://dashboard.gilas.io/apiKey>")),
10 base_url="https://api.gilas.io/v1/" # Gilas APIs
11)
12
13# Loading dataset
14dataset_path = "data/amazon_furniture_dataset.csv"
15df = pd.read_csv(dataset_path)
16df.head()
برچسب گذاری تصاویر #
در این بخش، ما از GPT-4V استفاده خواهیم کرد تا برچسب های مرتبط برای محصولات ایجاد کنیم. برای این کار از روش zero-shot برای استخراج کلیدواژه ها استفاده میکنیم، و با استفاده از embeddings این کلیدواژه ها را deduplicate خواهیم کرد تا از داشتن چندین کلیدواژه که خیلی شبیه یکدیگر هستند جلوگیری کنیم. برای جلوگیری از تولید برچسب برای دیگر آیتمهای موجود در عکس مثل لوازم دکوری داخل تصویر٬ از ترکیب تصاویر و عنوان محصولات استفاده خواهیم کرد تا از استخراج کلیدواژههای غیر مرتبط با موضوع اصلی تصویر جلوگیری کنیم.
توجه: ورودیهای داده شده و خروجیهای تولید شده توسط مدل در این مثال به زبان انگلیسی هستند. برای تولید خروجی به زبان فارسی٬ کافیست از مدل بخواهید که خروجی را به زبان فارسی تولید کند.
1system_prompt = '''
2 You are an agent specialized in tagging images of furniture items, decorative items, or furnishings with relevant keywords that could be used to search for these items on a marketplace.
3
4 You will be provided with an image and the title of the item that is depicted in the image, and your goal is to extract keywords for only the item specified.
5
6 Keywords should be concise and in lower case.
7
8 Keywords can describe things like:
9 - Item type e.g. 'sofa bed', 'chair', 'desk', 'plant'
10 - Item material e.g. 'wood', 'metal', 'fabric'
11 - Item style e.g. 'scandinavian', 'vintage', 'industrial'
12 - Item color e.g. 'red', 'blue', 'white'
13
14 Only deduce material, style or color keywords when it is obvious that they make the item depicted in the image stand out.
15
16 Return keywords in the format of an array of strings, like this:
17 ['desk', 'industrial', 'metal']
18
19'''
20
21def analyze_image(img_url, title):
22 response = client.chat.completions.create(
23 model="gpt-4-turbo",
24 messages=[
25 {
26 "role": "system",
27 "content": system_prompt
28 },
29 {
30 "role": "user",
31 "content": [
32 {
33 "type": "image_url",
34 "image_url": img_url,
35 },
36 ],
37 },
38 {
39 "role": "user",
40 "content": title
41 }
42 ],
43 max_tokens=300,
44 top_p=0.1
45 )
46
47 return response.choices[0].message.content
تست کردن نتیجه با چند تصویر مختلف
1examples = df.iloc[:5]
1for index, ex in examples.iterrows():
2 url = ex['primary_image']
3 img = Image(url=url)
4 display(img)
5 result = analyze_image(url, ex['title'])
6 print(result)
7 print("\n\n")
نتیجه:
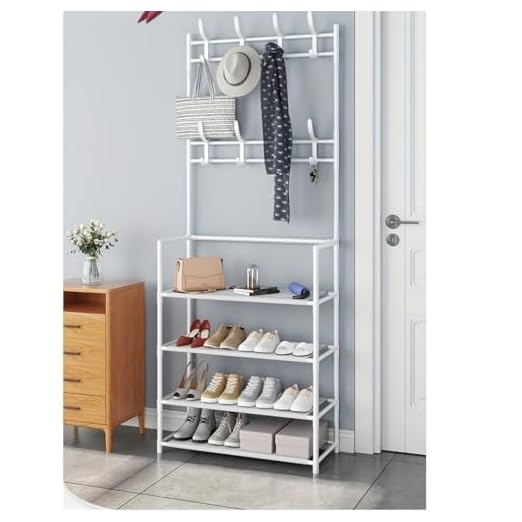
['shoe rack', 'free standing', 'multi-layer', 'metal', 'white']
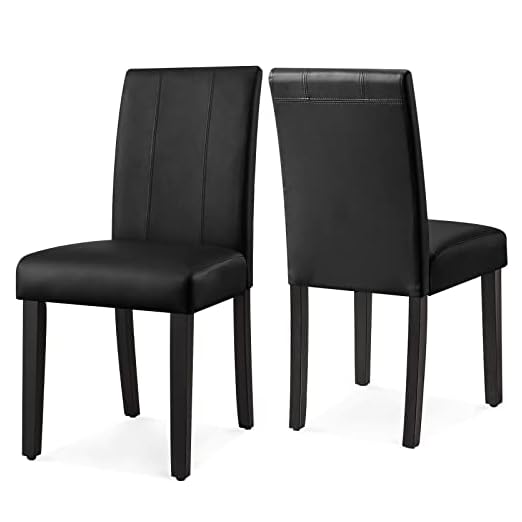
['dining chairs', 'set of 2', 'leather', 'black']
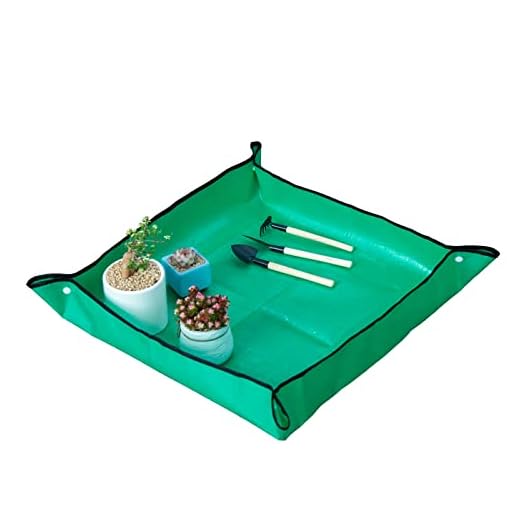
['plant repotting mat', 'waterproof', 'portable', 'foldable', 'easy to clean', 'green']
پیدا کردن برچسبهای مشابه #
برای پیدا و حذف کردن برچسبهای تکراری از embeddings استفاده میکنیم. embeddings روشی برای نمایش برداری متون است که معنا و مفهوم متن را شامل میشود.
1# Feel free to change the embedding model here
2def get_embedding(value, model="text-embedding-3-large"):
3 embeddings = client.embeddings.create(
4 model=model,
5 input=value,
6 encoding_format="float"
7 )
8 return embeddings.data[0].embedding
1df_keywords = pd.DataFrame(keywords_list, columns=['keyword'])
2df_keywords['embedding'] = df_keywords['keyword'].apply(lambda x: get_embedding(x))
3df_keywords
نمونه ای از نمایش برداری یک کلمه:
industrial [-0.026137426, 0.021297162, -0.007273361, -0.0...]
1def compare_keyword(keyword):
2 embedded_value = get_embedding(keyword)
3 df_keywords['similarity'] = df_keywords['embedding'].apply(lambda x: cosine_similarity(np.array(x).reshape(1,-1), np.array(embedded_value).reshape(1, -1)))
4 most_similar = df_keywords.sort_values('similarity', ascending=False).iloc[0]
5 return most_similar
6
7def replace_keyword(keyword, threshold = 0.6):
8 most_similar = compare_keyword(keyword)
9 if most_similar['similarity'] > threshold:
10 print(f"Replacing '{keyword}' with existing keyword: '{most_similar['keyword']}'")
11 return most_similar['keyword']
12 return keyword
1# Example keywords to compare to our list of existing keywords
2example_keywords = ['bed frame', 'wooden', 'vintage', 'old school', 'desk', 'table', 'old', 'metal', 'metallic', 'woody']
3final_keywords = []
4
5for k in example_keywords:
6 final_keywords.append(replace_keyword(k))
7
8final_keywords = set(final_keywords)
9print(f"Final keywords: {final_keywords}")
همانطور که در پایین مشاهده میکنید با استفاده از embeddings توانستیم برچسبهای مشابه را شناسایی کنیم:
Replacing 'bed frame' with existing keyword: 'bed'
Replacing 'wooden' with existing keyword: 'wood'
Replacing 'vintage' with existing keyword: 'vintage'
Replacing 'metal' with existing keyword: 'metal'
Replacing 'metallic' with existing keyword: 'metal'
Replacing 'woody' with existing keyword: 'wood'
Final keywords: {'table', 'desk', 'bed', 'old', 'vintage', 'metal', 'wood', 'old school'}
توضیح محتوای داخل یک عکس #
در این بخش، از GPT-4V برای تولید توضیحات تصویر استفاده خواهیم کرد و سپس از روش few-shot examples با GPT-4-turbo برای تولید عنوان برای هر تصویر استفاده خواهیم کرد. اگر روش زیر برای دیتابیس تصاویر شما به درستی عمل نمیکند میتوانید مدل را برای تصاویر خود fine-tune کنید.
1# Cleaning up dataset columns
2selected_columns = ['title', 'primary_image', 'style', 'material', 'color', 'url']
3df = df[selected_columns].copy()
حال از مدل GPT-4V برای توضیح محتوای تصاویر استفاده میکنیم:
1describe_system_prompt = '''
2 You are a system generating descriptions for furniture items, decorative items, or furnishings on an e-commerce website.
3 Provided with an image and a title, you will describe the main item that you see in the image, giving details but staying concise.
4 You can describe unambiguously what the item is and its material, color, and style if clearly identifiable.
5 If there are multiple items depicted, refer to the title to understand which item you should describe.
6 '''
7
8def describe_image(img_url, title):
9 response = client.chat.completions.create(
10 temperature=0.2,
11 messages=[
12 {
13 "role": "system",
14 "content": describe_system_prompt
15 },
16 {
17 "role": "user",
18 "content": [
19 {
20 "type": "image_url",
21 "image_url": img_url,
22 },
23 ],
24 },
25 {
26 "role": "user",
27 "content": title
28 }
29 ],
30 max_tokens=300,
31 )
32
33 return response.choices[0].message.content
نمونههایی از خروجی مدل روی تصاویر مختلف:
1for index, row in examples.iterrows():
2 print(f"{row['title'][:50]}{'...' if len(row['title']) > 50 else ''} - {row['url']} :\n")
3 img_description = describe_image(row['primary_image'], row['title'])
4 print(f"{img_description}\n--------------------------\n")
GOYMFK 1pc Free Standing Shoe Rack, Multi-layer Me... - https://www.amazon.com/dp/B0CJHKVG6P :
This is a free-standing shoe rack featuring a multi-layer design, constructed from metal for durability. The rack is finished in a clean white color, which gives it a modern and versatile look, suitable for various home decor styles. It includes several horizontal shelves dedicated to organizing shoes, providing ample space for multiple pairs.
--------------------------
subrtex Leather ding Room, Dining Chairs Set of 2,... - https://www.amazon.com/dp/B0B66QHB23 :
This image showcases a set of two dining chairs. The chairs are upholstered in black leather, featuring a sleek and modern design. They have a high back with subtle stitching details that create vertical lines, adding an element of elegance to the overall appearance. The legs of the chairs are also black, maintaining a consistent color scheme and enhancing the sophisticated look. These chairs would make a stylish addition to any contemporary dining room setting.
حال میتوانیم از توضیحات تولید شده برای تولید caption برای هر تصویر استفاده کنیم:
1caption_system_prompt = '''
2Your goal is to generate short, descriptive captions for images of furniture items, decorative items, or furnishings based on an image description.
3You will be provided with a description of an item image and you will output a caption that captures the most important information about the item.
4Your generated caption should be short (1 sentence), and include the most relevant information about the item.
5The most important information could be: the type of the item, the style (if mentioned), the material if especially relevant and any distinctive features.
6'''
7
8few_shot_examples = [
9 {
10 "description": "This is a multi-layer metal shoe rack featuring a free-standing design. It has a clean, white finish that gives it a modern and versatile look, suitable for various home decors. The rack includes several horizontal shelves dedicated to organizing shoes, providing ample space for multiple pairs. Above the shoe storage area, there are 8 double hooks arranged in two rows, offering additional functionality for hanging items such as hats, scarves, or bags. The overall structure is sleek and space-saving, making it an ideal choice for placement in living rooms, bathrooms, hallways, or entryways where efficient use of space is essential.",
11 "caption": "White metal free-standing shoe rack"
12 },
13 {
14 "description": "The image shows a set of two dining chairs in black. These chairs are upholstered in a leather-like material, giving them a sleek and sophisticated appearance. The design features straight lines with a slight curve at the top of the high backrest, which adds a touch of elegance. The chairs have a simple, vertical stitching detail on the backrest, providing a subtle decorative element. The legs are also black, creating a uniform look that would complement a contemporary dining room setting. The chairs appear to be designed for comfort and style, suitable for both casual and formal dining environments.",
15 "caption": "Set of 2 modern black leather dining chairs"
16 },
17 {
18 "description": "This is a square plant repotting mat designed for indoor gardening tasks such as transplanting and changing soil for plants. It measures 26.8 inches by 26.8 inches and is made from a waterproof material, which appears to be a durable, easy-to-clean fabric in a vibrant green color. The edges of the mat are raised with integrated corner loops, likely to keep soil and water contained during gardening activities. The mat is foldable, enhancing its portability, and can be used as a protective surface for various gardening projects, including working with succulents. It's a practical accessory for garden enthusiasts and makes for a thoughtful gift for those who enjoy indoor plant care.",
19 "caption": "Waterproof square plant repotting mat"
20 }
21]
22
23formatted_examples = [[{
24 "role": "user",
25 "content": ex['description']
26},
27{
28 "role": "assistant",
29 "content": ex['caption']
30}]
31 for ex in few_shot_examples
32]
33
34formatted_examples = [i for ex in formatted_examples for i in ex]
1def caption_image(description, model="gpt-4-turbo"):
2 messages = formatted_examples
3 messages.insert(0,
4 {
5 "role": "system",
6 "content": caption_system_prompt
7 })
8 messages.append(
9 {
10 "role": "user",
11 "content": description
12 })
13 response = client.chat.completions.create(
14 model=model,
15 temperature=0.2,
16 messages=messages
17 )
18
19 return response.choices[0].message.content
نمونههایی از خروجی مدل روی تصاویر مختلف:
1examples = df.iloc[5:8]
2for index, row in examples.iterrows():
3 print(f"{row['title'][:50]}{'...' if len(row['title']) > 50 else ''} - {row['url']} :\n")
4 img_description = describe_image(row['primary_image'], row['title'])
5 print(f"{img_description}\n--------------------------\n")
6 img_caption = caption_image(img_description)
7 print(f"{img_caption}\n--------------------------\n")
LOVMOR 30'' Bathroom Vanity Sink Base Cabine, Stor... - https://www.amazon.com/dp/B0C9WYYFLB:
Image description:
This is a LOVMOR 30'' Bathroom Vanity Sink Base Cabinet featuring a classic design with a rich brown finish. The cabinet is designed to provide ample storage with three drawers on the left side, offering organized space for bathroom essentials...
--------------------------
Short caption:
LOVMOR 30'' classic brown bathroom vanity base cabinet with three drawers and additional storage space.
--------------------------
Folews Bathroom Organizer Over The Toilet Storage,... - https://www.amazon.com/dp/B09NZY3R1T :
Image description:
This is a 4-tier bathroom organizer designed to fit over a standard toilet, providing a space-saving storage solution. The unit is constructed with a sturdy metal frame in a black finish, which offers both durability and a sleek, modern look....
--------------------------
Short caption:
Modern 4-tier black metal bathroom organizer with adjustable shelves and baskets, designed to fit over a standard toilet for space-saving storage.
--------------------------
جستجو میان تصاویر #
در این مرحله از کلمات کلیدی و عناوین تولید شده برای جستجوی میان تصاویر بر اساس متن یا تصویر استفاده خواهیم کرد.
ما از مدل embeddings خود به منظور تولید بردارها برای کلمات کلیدی و عناوین استفاده خواهیم کرد و آنها را با متن ورودی یا عنوان تولید شده از یک تصویر ورودی مقایسه خواهیم کرد. توجه داشته باشید که اگر ورودی یک تصویر باشد ابتدا با استفاده از روشهای بالا آن تصویر را به صورت متنی توصیف میکنیم و سپس بر اساس آن توصیف دیتابیس را برای پیدا کردن تصاویر مشابه جستجو میکنیم.
1# Df we'll use to compare keywords
2df_keywords = pd.DataFrame(columns=['keyword', 'embedding'])
3df['keywords'] = ''
4df['img_description'] = ''
5df['caption'] = ''
1# Function to replace a keyword with an existing keyword if it's too similar
2def get_keyword(keyword, df_keywords, threshold = 0.6):
3 embedded_value = get_embedding(keyword)
4 df_keywords['similarity'] = df_keywords['embedding'].apply(lambda x: cosine_similarity(np.array(x).reshape(1,-1), np.array(embedded_value).reshape(1, -1)))
5 sorted_keywords = df_keywords.copy().sort_values('similarity', ascending=False)
6 if len(sorted_keywords) > 0 :
7 most_similar = sorted_keywords.iloc[0]
8 if most_similar['similarity'] > threshold:
9 print(f"Replacing '{keyword}' with existing keyword: '{most_similar['keyword']}'")
10 return most_similar['keyword']
11 new_keyword = {
12 'keyword': keyword,
13 'embedding': embedded_value
14 }
15 df_keywords = pd.concat([df_keywords, pd.DataFrame([new_keyword])], ignore_index=True)
16 return keyword
آماده کردن دیتاست #
1import ast
2
3def tag_and_caption(row):
4 keywords = analyze_image(row['primary_image'], row['title'])
5 try:
6 keywords = ast.literal_eval(keywords)
7 mapped_keywords = [get_keyword(k, df_keywords) for k in keywords]
8 except Exception as e:
9 print(f"Error parsing keywords: {keywords}")
10 mapped_keywords = []
11 img_description = describe_image(row['primary_image'], row['title'])
12 caption = caption_image(img_description)
13 return {
14 'keywords': mapped_keywords,
15 'img_description': img_description,
16 'caption': caption
17 }
پردازش همهٔ ۳۱۲ خط مجموعه داده مدتی زمان میبرد. برای آزمایش این ایده، فقط آن را بر روی اولین ۵۰ خط اجرا خواهیم کرد که تقریباً ۲۰ دقیقه زمان میبرد. اگر تمایل دارید این مرحله را انجام ندهید و مجموعه داده از پیش پردازش شده را بارگذاری کنید جدول پایین را مشاهده کنید.
title | primary_image | style | material | color | url | keywords | img_description | caption | |
---|---|---|---|---|---|---|---|---|---|
0 | GOYMFK 1pc Free Standing Shoe Rack, Multi-laye... | https://m.media-amazon.com/images/I/416WaLx10j... | Modern | Metal | White | https://www.amazon.com/dp/B0CJHKVG6P | [shoe rack, free standing, multi-layer, metal,... | This is a free-standing shoe rack featuring a ... | White metal free-standing shoe rack with multi... |
1 | subrtex Leather ding Room, Dining Chairs Set o... | https://m.media-amazon.com/images/I/31SejUEWY7... | Black Rubber Wood | Sponge | Black | https://www.amazon.com/dp/B0B66QHB23 | [dining chairs, set of 2, leather, black] | This image features a set of two black dining ... | Set of 2 sleek black faux leather dining chair... |
2 | Plant Repotting Mat MUYETOL Waterproof Transpl... | https://m.media-amazon.com/images/I/41RgefVq70... | Modern | Polyethylene | Green | https://www.amazon.com/dp/B0BXRTWLYK | [plant repotting mat, waterproof, portable, fo... | This is a square plant repotting mat designed ... | Waterproof green square plant repotting mat |
3 | Pickleball Doormat, Welcome Doormat Absorbent ... | https://m.media-amazon.com/images/I/61vz1Igler... | Modern | Rubber | A5589 | https://www.amazon.com/dp/B0C1MRB2M8 | [doormat, absorbent, non-slip, brown] | This is a rectangular doormat featuring a play... | Pickleball-themed coir doormat with playful de... |
4 | JOIN IRON Foldable TV Trays for Eating Set of ... | https://m.media-amazon.com/images/I/41p4d4VJnN... | X Classic Style | Iron | Grey Set of 4 | https://www.amazon.com/dp/B0CG1N9QRC | [tv tray table set, foldable, iron, grey] | This image showcases a set of two foldable TV ... | Set of two foldable TV trays with grey wood gr... |
1# Saving locally for later
2data_path = "data/items_tagged_and_captioned.csv"
3df.to_csv(data_path, index=False)
4
5# Optional: load data from saved file
6df = pd.read_csv(data_path)
تولید embeddings برای برچسبها و کپشن تصاویر #
اکنون میتوانیم از عناوین و کلمات کلیدی تولید شده برای همسانسازی محتوای مرتبط برای جستجوی متن ورودی یا عنوان استفاده کنیم. برای انجام این کار، یک ترکیب از کلمات کلیدی + عناوین را تهیه خواهیم کرد.
ایجاد embeddings حدود ۳ دقیقه زمان میبرد. اگر تمایل دارید، میتوانید مجموعه داده از پیش پردازش شده را بارگذاری کنید.
1df_search = df.copy()
2
3def embed_tags_caption(x):
4 if x['caption'] != '':
5 keywords_string = ",".join(k for k in x['keywords']) + '\n'
6 content = keywords_string + x['caption']
7 embedding = get_embedding(content)
8 return embedding
9
10df_search['embedding'] = df_search.apply(lambda x: embed_tags_caption(x), axis=1)
11
12df_search.head()
title | primary_image | style | material | color | url | keywords | img_description | caption | embedding | |
---|---|---|---|---|---|---|---|---|---|---|
0 | GOYMFK 1pc Free Standing Shoe Rack, Multi-laye... | https://m.media-amazon.com/images/I/416WaLx10j... | Modern | Metal | White | https://www.amazon.com/dp/B0CJHKVG6P | ['shoe rack', 'free standing', 'multi-layer', ... | This is a free-standing shoe rack featuring a ... | White metal free-standing shoe rack with multi... | [-0.06596625, -0.026769113, -0.013789515, -0.0... |
1 | subrtex Leather ding Room, Dining Chairs Set o... | https://m.media-amazon.com/images/I/31SejUEWY7... | Black Rubber Wood | Sponge | Black | https://www.amazon.com/dp/B0B66QHB23 | ['dining chairs', 'set of 2', 'leather', 'black'] | This image features a set of two black dining ... | Set of 2 sleek black faux leather dining chair... | [-0.0077859573, -0.010376813, -0.01928079, -0.... |
2 | Plant Repotting Mat MUYETOL Waterproof Transpl... | https://m.media-amazon.com/images/I/41RgefVq70... | Modern | Polyethylene | Green | https://www.amazon.com/dp/B0BXRTWLYK | ['plant repotting mat', 'waterproof', 'portabl... | This is a square plant repotting mat designed ... | Waterproof green square plant repotting mat | [-0.023248248, 0.005370147, -0.0048999498, -0.... |
3 | Pickleball Doormat, Welcome Doormat Absorbent ... | https://m.media-amazon.com/images/I/61vz1Igler... | Modern | Rubber | A5589 | https://www.amazon.com/dp/B0C1MRB2M8 | ['doormat', 'absorbent', 'non-slip', 'brown'] | This is a rectangular doormat featuring a play... | Pickleball-themed coir doormat with playful de... | [-0.028953036, -0.026369056, -0.011363288, 0.0... |
4 | JOIN IRON Foldable TV Trays for Eating Set of ... | https://m.media-amazon.com/images/I/41p4d4VJnN... | X Classic Style | Iron | Grey Set of 4 | https://www.amazon.com/dp/B0CG1N9QRC | ['tv tray table set', 'foldable', 'iron', 'grey'] | This image showcases a set of two foldable TV ... | Set of two foldable TV trays with grey wood gr... | [-0.030723095, -0.0051356032, -0.027088132, 0.... |
1# Keep only the lines where we have embeddings
2df_search = df_search.dropna(subset=['embedding'])
3print(df_search.shape)
4
5(49, 10)
1# Saving locally for later
2data_embeddings_path = "data/items_tagged_and_captioned_embeddings.csv"
3df_search.to_csv(data_embeddings_path, index=False)
4
5# Optional: load data from saved file
6from ast import literal_eval
7df_search = pd.read_csv(data_embeddings_path)
8df_search["embedding"] = df_search.embedding.apply(literal_eval).apply(np.array)
جستجوی بر اساس متن ورودی #
برای جستجوی میان تصاویر بر اساس متن ورودی میتوانیم embeddings منن ورودی را تولید کرده و آن را با embeddings تولید شده برای تصاویر که در مراحل قبل تولید کردهایم مقایسه کنیم.
1# Searching for N most similar results
2def search_from_input_text(query, n = 2):
3 embedded_value = get_embedding(query)
4 df_search['similarity'] = df_search['embedding'].apply(lambda x: cosine_similarity(np.array(x).reshape(1,-1), np.array(embedded_value).reshape(1, -1)))
5 most_similar = df_search.sort_values('similarity', ascending=False).iloc[:n]
6 return most_similar
7
8user_inputs = ['shoe storage', 'black metal side table', 'doormat', 'step bookshelf', 'ottoman']
9
10for i in user_inputs:
11 print(f"Input: {i}\n")
12 res = search_from_input_text(i)
13 for index, row in res.iterrows():
14 similarity_score = row['similarity']
15 if isinstance(similarity_score, np.ndarray):
16 similarity_score = similarity_score[0][0]
17 print(f"{row['title'][:50]}{'...' if len(row['title']) > 50 else ''} ({row['url']}) - Similarity: {similarity_score:.2f}")
18 img = Image(url=row['primary_image'])
19 display(img)
20 print("\n\n")
#متن ورودی برای جستجو
Input: shoe storage
# تصویر متناسب با ورودی
GOYMFK 1pc Free Standing Shoe Rack, Multi-layer Me... (https://www.amazon.com/dp/B0CJHKVG6P) - Similarity: 0.62
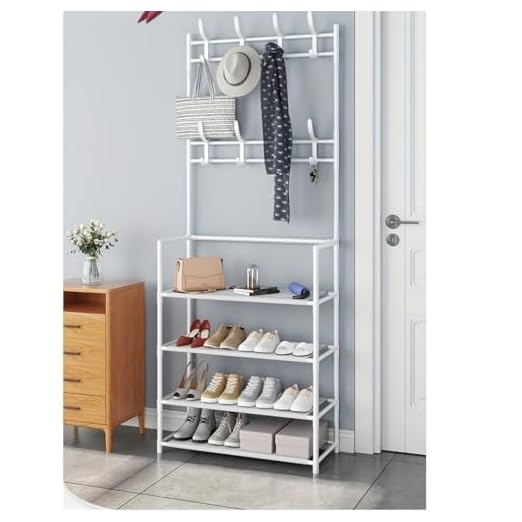
جستجوی بر اساس تصویر ورودی #
اگر ورودی یک تصویر باشد، میتوانیم برای یافتن تصاویر مشابه ابتدا برای تصویر ورودی عنوان و برچسب تولید میکنیم٬ سپس برای آنها embeddings میسازیم و نهایتا بر اساس embeddings های تولید شده در میان دیتابیس جستجو میکنیم.
1# We'll take a mix of images: some we haven't seen and some that are already in the dataset
2example_images = df.iloc[306:]['primary_image'].to_list() + df.iloc[5:10]['primary_image'].to_list()
3
4for i in example_images:
5 img_description = describe_image(i, '')
6 caption = caption_image(img_description)
7 img = Image(url=i)
8 print('Input: \n')
9 display(img)
10 res = search_from_input_text(caption, 1).iloc[0]
11 similarity_score = res['similarity']
12 if isinstance(similarity_score, np.ndarray):
13 similarity_score = similarity_score[0][0]
14 print(f"{res['title'][:50]}{'...' if len(res['title']) > 50 else ''} ({res['url']}) - Similarity: {similarity_score:.2f}")
15 img_res = Image(url=res['primary_image'])
16 display(img_res)
17 print("\n\n")
18
ورودی:
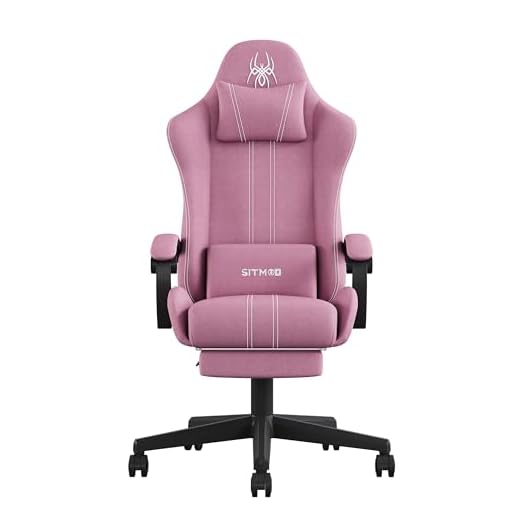
خروجی:
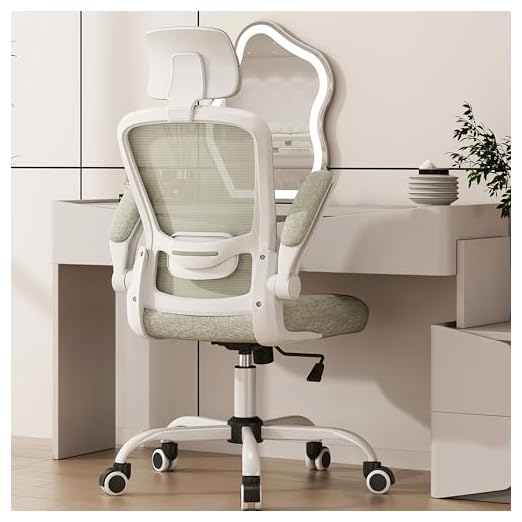
جمع بندی #
در این نوتبوک، ما بررسی کردیم که چگونه میتوانیم از قابلیتهای GPT-4V برای برچسب زدن و توضیح دادن تصاویر استفاده کنیم. با ارائه تصاویر همراه با اطلاعات مرتبط به مدل، توانستیم تگها و توضیحاتی تولید کنیم که میتوانند با استفاده از یک مدل زبانی مانند GPT-4-turbo بیشتر پالایش شده و برای ایجاد توضیحات استفاده شوند. این فرآیند کاربردهای عملی مختلفی دارد، به ویژه در بهبود عملکرد جستجو.